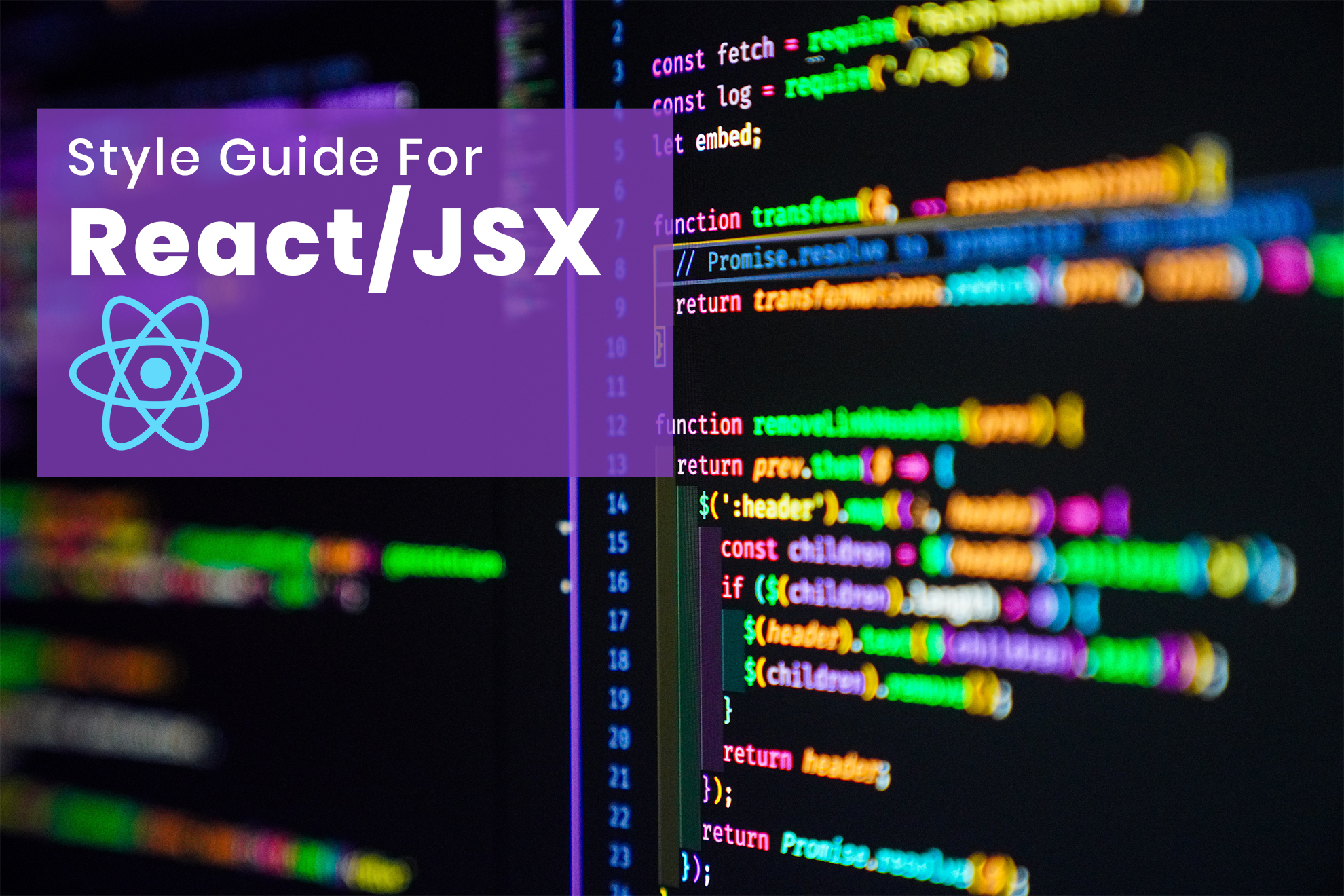
Style guides are written so that new developers may quickly become comfortable with a code base and then generate code that other developers can understand.
TABLE OF CONTENTS
-
Basic Rules
-
Naming
-
Declaration
-
Alignment
-
Props
-
Refs
-
Tags
-
Methods
-
Ordering
-
Code Style Best Practices
Basic Rules
- Each file should only include one React component. Multiple Stateless, or Pure, Components are, however, permitted per file
- Use the JSX syntax at all times.
- Do not use React.createElement unless you’re initializing the app from a file that is not JSX.
Naming
Extensions: Use
.jsx
. extension for React components.
Filename: Use PascalCase for filenames. E.g.,
ReservationCard.jsx
.
Reference Naming: Use PascalCase for React components and camelCase for their instances. eslint:
ReservationCard.jsx
.
Component Naming: Use the filename as the component name. For example, ReservationCard.jsx . should have a reference name of ReservationCard . However, for root components of a directory, use index.jsx as the filename and use the directory name as the component name
Declaration
Do not use displayName for naming components. Instead, name the component by reference.
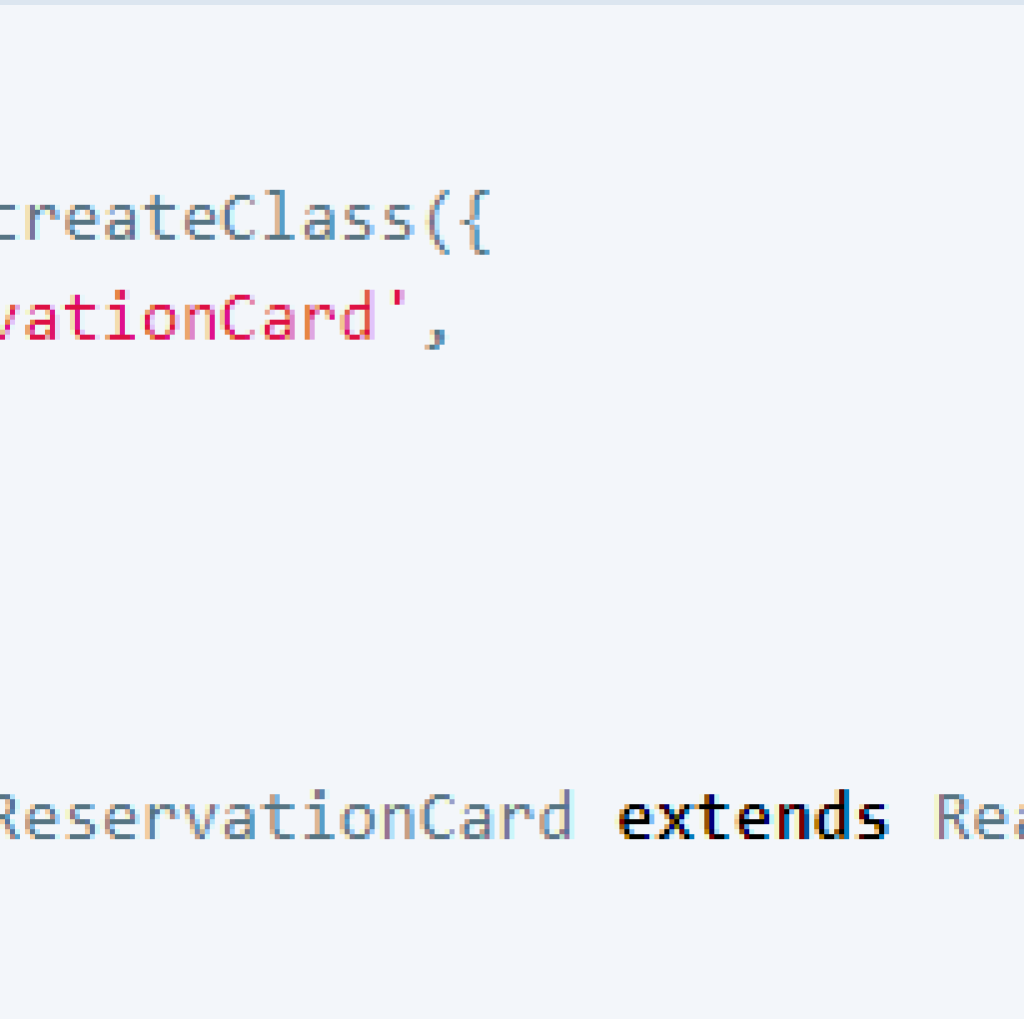
Alignment
Follow these alignment styles for JSX syntax. eslint: react/jsx-closing-bracket-location react/jsx-closing-tag-location
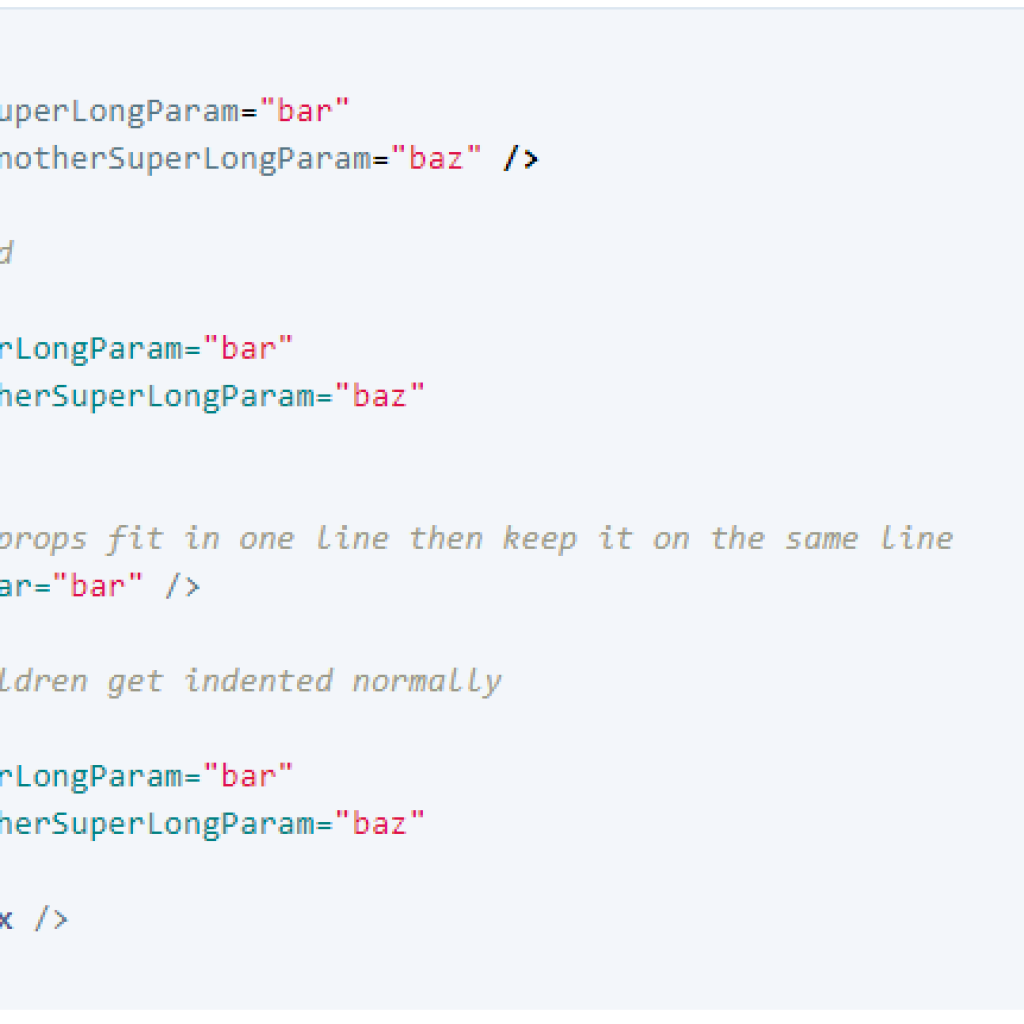
Props
Always use camelCase for prop names.
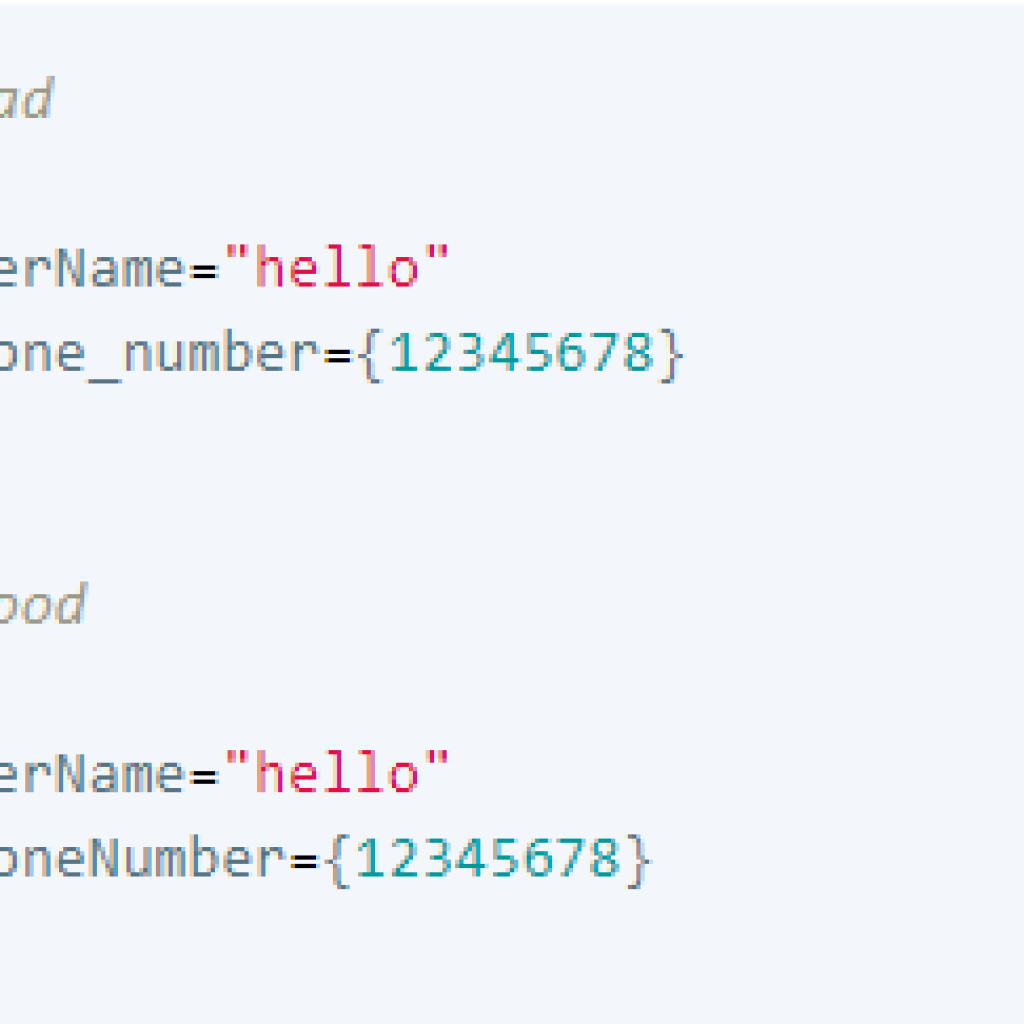
Do not use words like “image”, “photo”, or “picture” in
alt
props. eslint:
jsx-a11y/img-redundant-alt
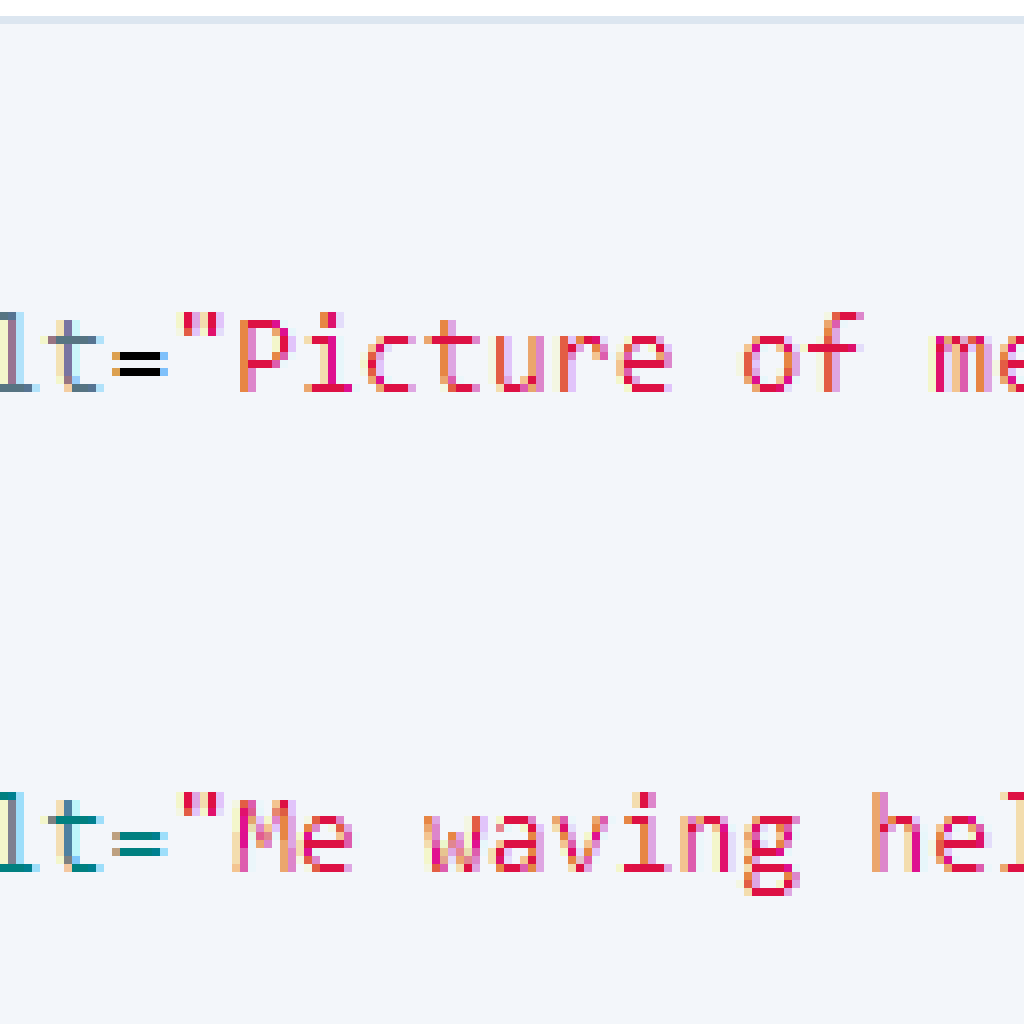
Avoid using an array index as key prop, prefer a unique ID.
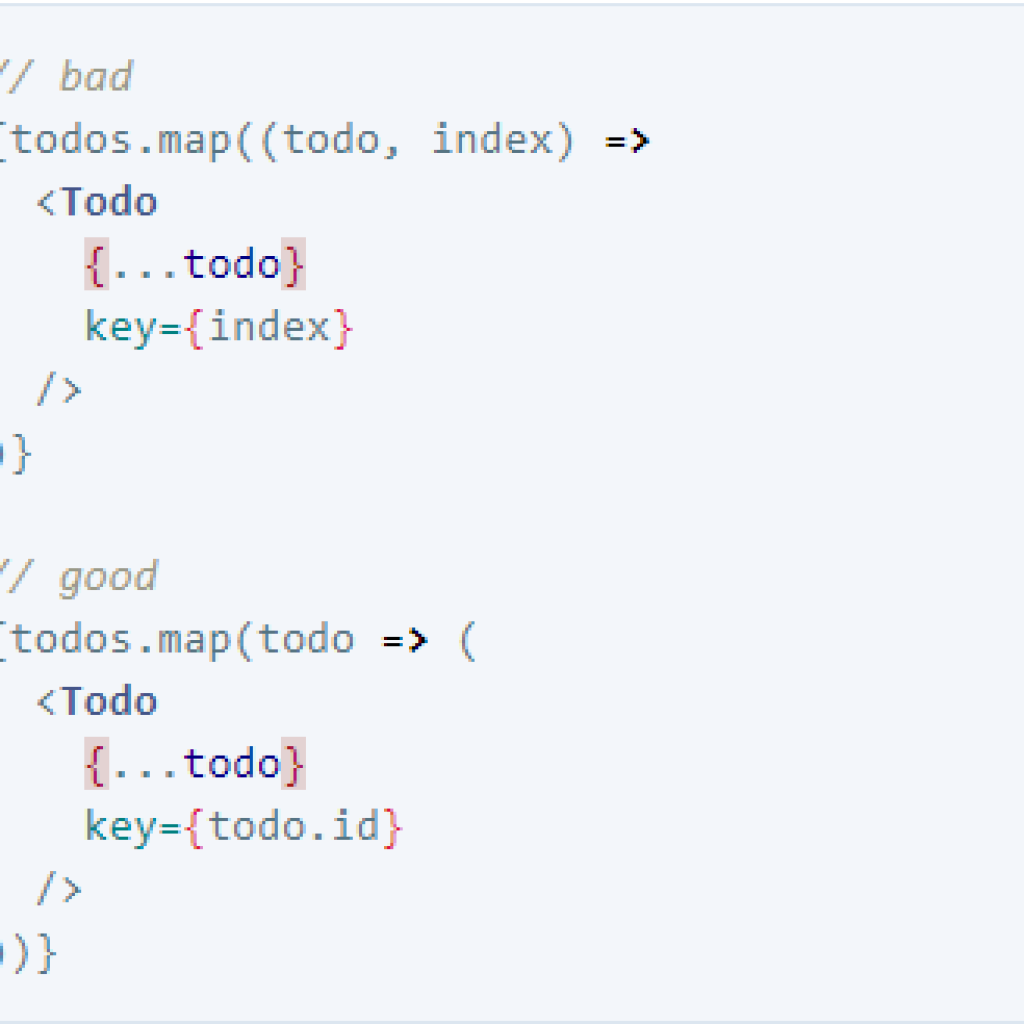
Spreading objects with known, explicit props. This can be particularly useful when testing React components with Mocha’s beforeEach construct.
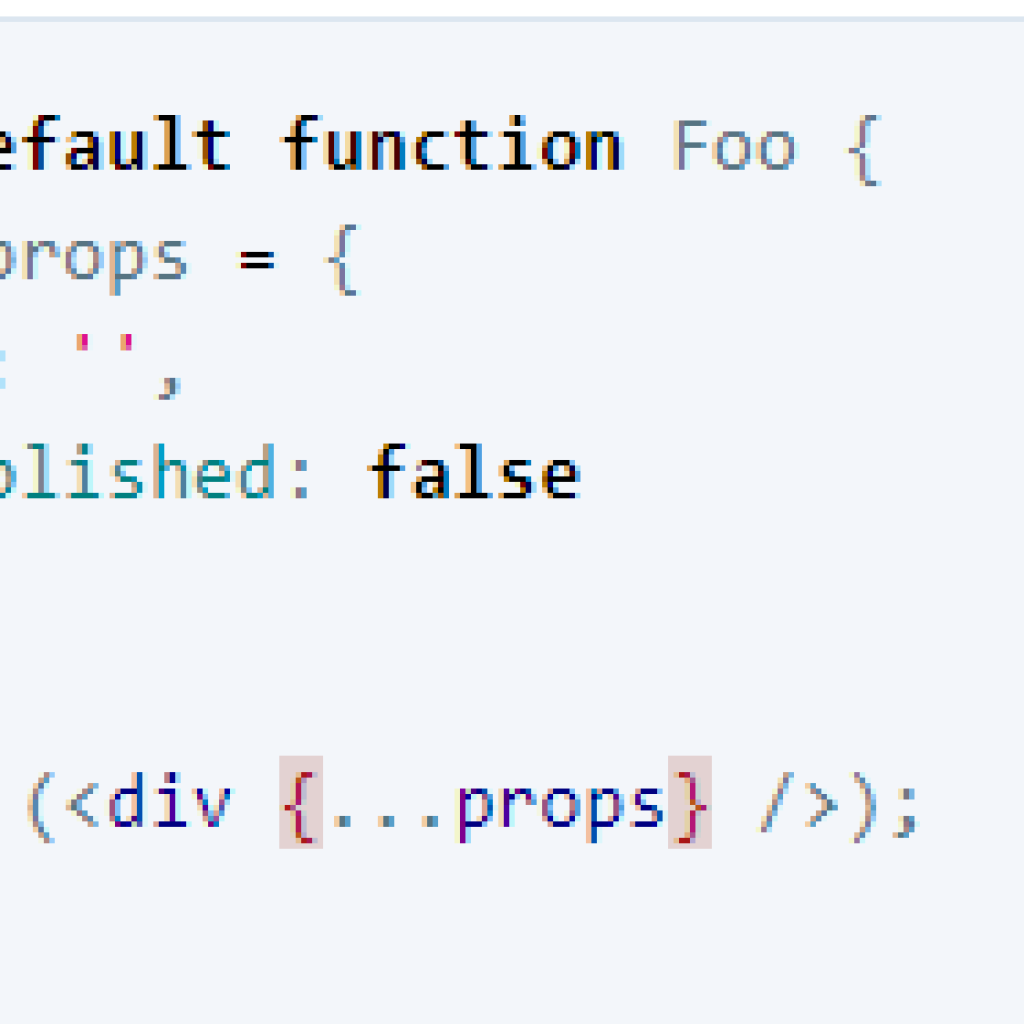
Refs
Always use ref callbacks. eslint: react/no-string-refs
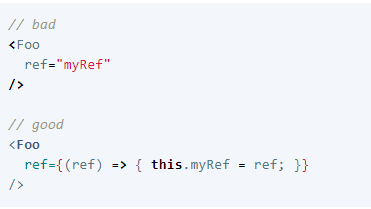
Tags
Always self-close tags that have no children. eslint: react/self-closing-comp
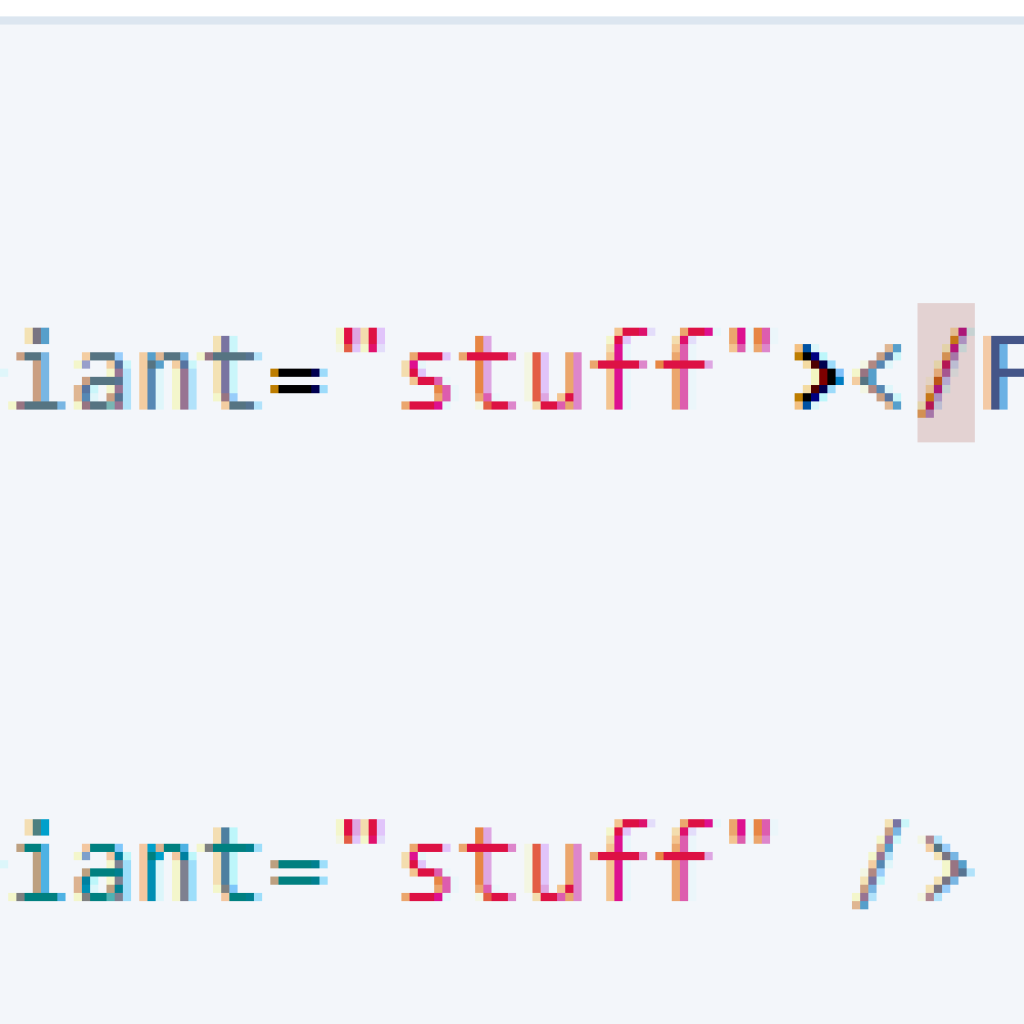
If your component has multi-line properties, close its tag on a new line. eslint: react/jsx-closing-bracket-location
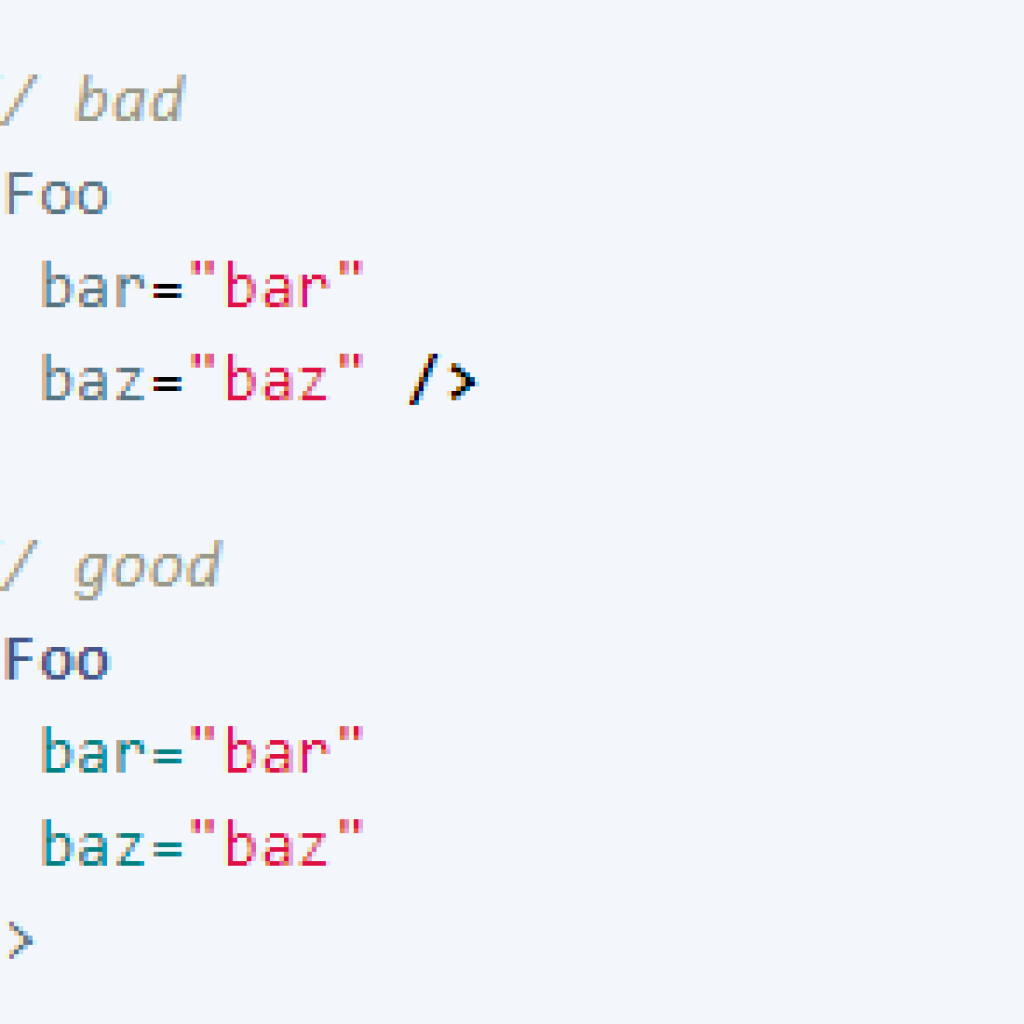
Methods
Use arrow functions to close over local variables.
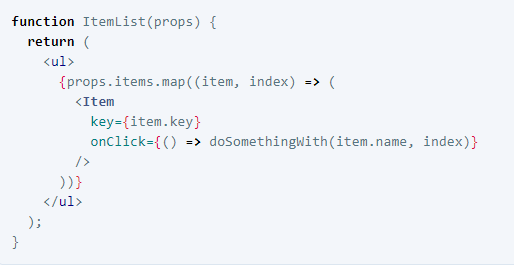
Do not use underscore prefix for internal methods of a React component
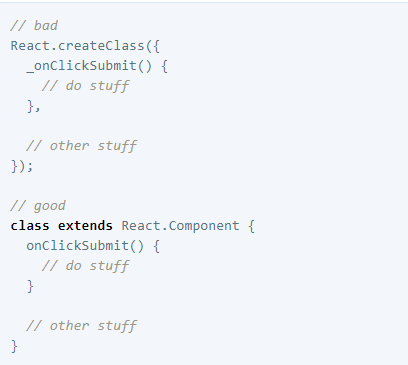
Ordering
Ordering for class extends React.Component:
- optional static methods
- constructor
- getChildContext
- componentWillMount
- componentDidMount
- componentWillReceiveProps
- shouldComponentUpdate
- optional static methods
- constructor
- getChildContext
- componentWillMount
- componentDidMount
- componentWillReceiveProps
- shouldComponentUpdate
Code Style Best Practices
- Avoid the Use of the State as much as Possible
- Whenever using state in the component, keep it centralized to that component and pass it down in the component tree as props.
- Write DRY Code
- Try to avoid duplicate code and create a common component to perform the repetitive task to maintain the DRY (Don’t Repeat Yourself) code structure.
- For instance: When you need to show multiple buttons on a screen then you can create a common button component and use it rather than writing markup for each button
- Remove Unnecessary Comments from the Code
- Use Destructuring to Get Props
-
- Destructuring was introduced in ES6. This type of feature in the javascript function allows you to easily extract the form data and assign your variables from the object or array. Also, destructuring props make code cleaner and easier to read.
-
- Apply ES6 Spread Function
- It would be a more easy and productive way to use ES6 functions to pass an object property. Using {…props} between the open and close tag will automatically insert all the props of the object.
- Manage too many Props with Parent/Child Component
-
- Use Map Function for Dynamic Rendering of Arrays
- Use es-lint or Prettier for Formatting
Conclusion
With this blog of React/JSX style guide, we gave you a deeper insight for react js developer and frontend developer on how ReactJS works. The React best practices will offer you fewer typing options and more explicit codes. Once you will start using this, you will start liking its clear crisp features with code reusability. This list of best practices from React will help you place your ventures on the right path, and later down the line, eliminate any future development complications.